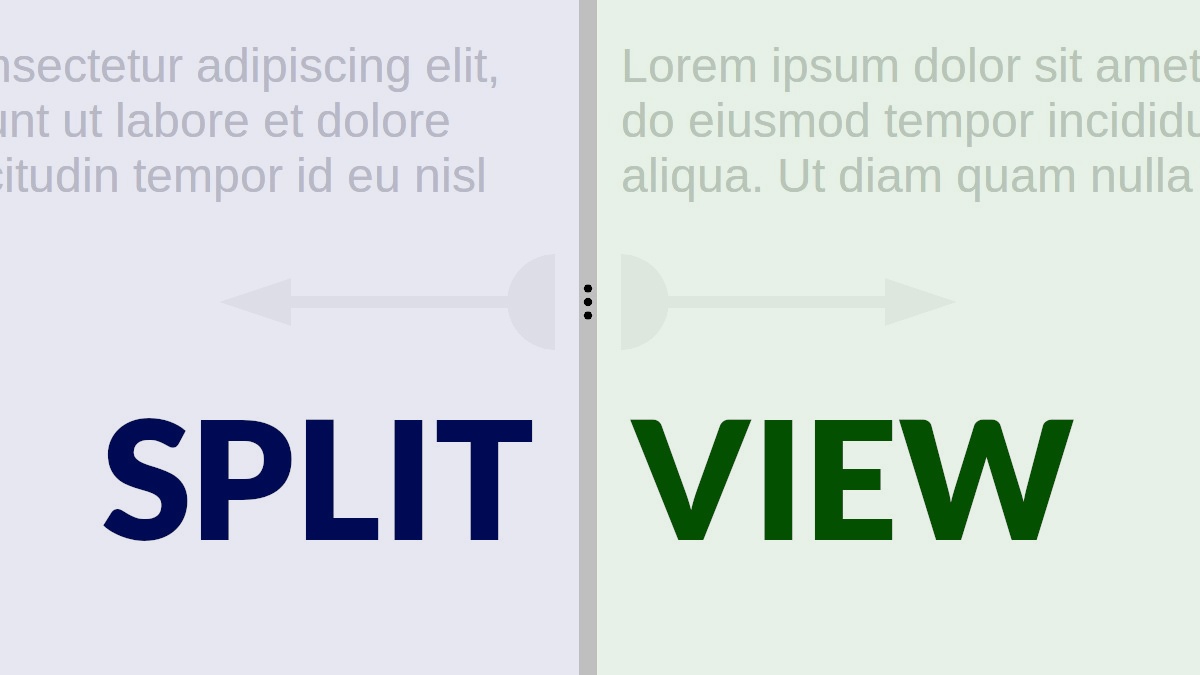
Resizable Split View
A Fast, lightweight and easy to use container for two resizable panes(frames), supports minimum size boundaries.JavaScript, HTML, SCSS Download (1KB): split-view.min.jsDemo
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Leo a diam sollicitudin tempor id eu nisl nunc.
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut diam quam nulla porttitor massa id.
HTML / JS
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
<!DOCTYPE html>
<html>
<head>
...
<script src="/path/to/split-view.js"></script>
...
</head>
<body>
...
<div id="wrap_id" class="split-wrap">
<div id="left_id" class="split-left">
<!-- Insert Your Element(s) Here -->
</div>
<div id="handle_id" class="split-handle"></div>
<div id="right_id" class="split-right">
<!-- Insert Your Element(s) Here -->
</div>
</div>
<script>
document.getElementById("sid_wrap").split_view=new SplitView("left_id","handle_id","right_id")
</script>
...
</body>
</html>
SCSS
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
$split_handle_width:6px;
$split_original_position:20%;
$split_background_color: #d3d3d3;
.split-wrap{
position: relative;
border: thick solid $split_background_color;
}
.split-left,
.split-handle,
.split-right{
position: absolute;
display: block;
height: 100%;
top:0;
padding: 0;
margin: 0;
box-sizing: border-box;
overflow: auto;
}
.split-left{
left: 0;
width: $split_original_position
}
.split-handle{
left: $split_original_position;
width: $split_handle_width;
background-image: url("data:image/svg+xml;base64,PHN2ZyB2ZXJzaW9uPSIxLjEiIHdpZHRoPSI2IiBoZWlnaHQ9IjE2IiB2aWV3Qm94PSIwIDAgNiAxNiIgeG1sbnM9Imh0dHA6Ly93d3cudzMub3JnLzIwMDAvc3ZnIj48cGF0aCBmaWxsPSIjNzc3IiBkPSJtMyAxMWExLjUgMS41IDAgMCAxIDEuNSAxLjUgMS41IDEuNSAwIDAgMS0xLjUgMS41IDEuNSAxLjUgMCAwIDEtMS41LTEuNSAxLjUgMS41IDAgMCAxIDEuNS0xLjVtMC00LjVhMS41IDEuNSAwIDAgMSAxLjUgMS41IDEuNSAxLjUgMCAwIDEtMS41IDEuNSAxLjUgMS41IDAgMCAxLTEuNS0xLjUgMS41IDEuNSAwIDAgMSAxLjUtMS41bTAtNC41YTEuNSAxLjUgMCAwIDEgMS41IDEuNSAxLjUgMS41IDAgMCAxLTEuNSAxLjUgMS41IDEuNSAwIDAgMS0xLjUtMS41IDEuNSAxLjUgMCAwIDEgMS41LTEuNXoiLz48L3N2Zz4=");
background-repeat: no-repeat;
background-position: center;
background-color: $split_background_color;
cursor: col-resize;
z-index: 4;
}
.split-right{
right: 0;
left: $split_original_position;
margin-left: $split_handle_width;
}
.split-cursor-resize{
cursor: col-resize;
}
split-view.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
"use strict"
/**
* @implements {EventListener}
* @constructor
* @param {string} leftId
* @param {string} handleId
* @param {string} rightId
* @param {number} [leftMin=0] - Left side min. width in percent
* @param {number} [rightMin=0] - Right side min. width in percent
*/
function SplitView(leftId,handleId,rightId,leftMin,rightMin) {
/**
* @type {Element|undefined}
* @private
**/
this.p = undefined
/** @private **/
this.x = 0
/** @private **/
this.lMin = 1
/** @private **/
this.rMin = 98
/** @private **/
this.drag = false
/** @private **/
this.lastPr = 0 | 0
/** @private **/
this.lof = 0
/** @private **/
this.h=document.getElementById(handleId)
/** @private **/
this.l=document.getElementById(leftId)
/** @private **/
this.r=document.getElementById(rightId)
this.hw=this.h.getBoundingClientRect().width
if(leftMin && leftMin>0){
this.lMin=leftMin
this.lastPr=leftMin
this.l.style.width=this.r.style.left=this.h.style.left=leftMin+"%"
}
if(rightMin && rightMin>0) this.rMin=100-rightMin
this.h.addEventListener('mousedown',this,false)
this.p=this.h.parentElement
}
/**
* @private
* @param {Event|MouseEvent} e
* */
SplitView.prototype.handleEvent=function(e){
var pr
switch (e.type) {
case 'mousedown':
this.drag=true
this.p.addEventListener('mousemove',this,false)
// "window" is because we can end up outside of the parent
window.addEventListener('mouseup',this,false)
this.opc=this.p.className
this.p.className+=" split-cursor-resize"
this.lof=this.p.getBoundingClientRect().left+this.hw
e.preventDefault()
break
case 'mousemove':
if(this.drag===true){
pr=((e.clientX-this.lof)/this.p.clientWidth*100)|0
if(pr!==this.lastPr && (pr>=this.lMin && pr<=this.rMin)) {
this.lastPr=pr
this.l.style.width=this.r.style.left=this.h.style.left=pr+"%"
}
}
break
case 'mouseup':
if(this.drag===false) return
this.drag=false
this.p.removeEventListener('mousemove',this,false)
window.removeEventListener('mouseup',this,false)
this.p.className=this.opc
break
}
}