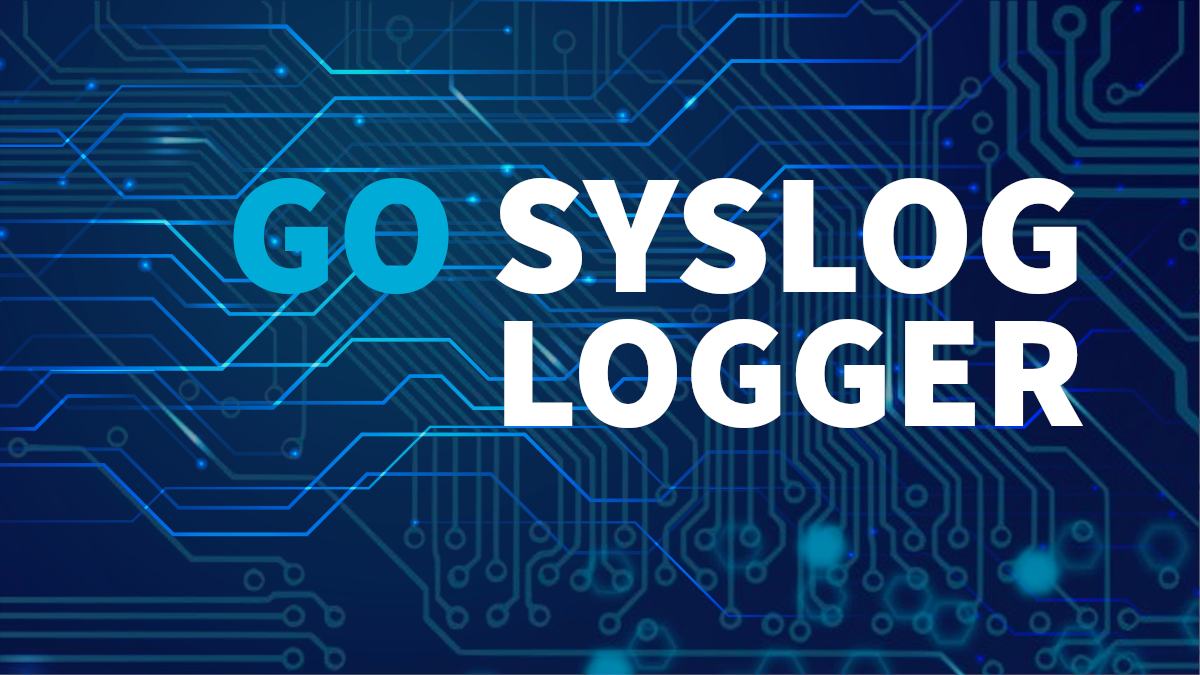
Golang Syslog Logger
A very simple Go logger with support for multiple syslog/journal severity levels. Intended for apps/services started via systemd and properly set StandardOutput & SyslogIdentifier values.Golang, Syslog, systemd Download: logsl.goAbout
Simplicity is the primary goal of this package, it supports 3 severity levels in addition to Panic and Fatal functions. Although, it can be used on its own (without systemd) to write to STDOUT, it really is designed to take advantage of systemd's StandardOutput=, SyslogIdentifier= and SyslogFacility= directives.
By default the StandardOutput= value of systemd units is set to journal, and any external, additional syslog daemons receive their log data from the journal, too, hence this is the option to use when logging shall be processed with such a daemon. Logging prefixes (severity levels) are defined as macros in sd-daemon.h and can be used by simply prefixing output lines with one of the strings.
systemd/sd-daemon.h
...
#define SD_EMERG "<0>" /* system is unusable */
#define SD_ALERT "<1>" /* action must be taken immediately */
#define SD_CRIT "<2>" /* critical conditions */
#define SD_ERR "<3>" /* error conditions */
#define SD_WARNING "<4>" /* warning conditions */
#define SD_NOTICE "<5>" /* normal but significant condition */
#define SD_INFO "<6>" /* informational */
#define SD_DEBUG "<7>" /* debug-level messages */
...
Testing with systemd
Given the logsl-test.go test program started via logsl_test.service systemd unit, the logs will look similar to journal output below:
journalctl -u logsl_test
Aug 01 12:36:04 archlnx systemd[1]: Started Logsl TEST. Aug 01 12:36:04 archlnx logsl_test[4216]: Hello, I am Info Aug 01 12:36:04 archlnx logsl_test[4216]: WARNING: Hello, I am Warning Aug 01 12:36:04 archlnx logsl_test[4216]: ERROR: Hello, I am Error Aug 01 12:36:04 archlnx logsl_test[4216]: FATAL: Hello, I am Fatal Aug 01 12:36:04 archlnx systemd[1]: logsl_test.service: Main process exited, code=exited, status=1/FAILURE Aug 01 12:36:04 archlnx systemd[1]: logsl_test.service: Failed with result 'exit-code'.
logsl_test.service
1
2
3
4
5
6
7
8
9
10
11
12
13
[Unit]
Description=Logsl TEST
[Service]
Type=simple
ExecStart=/absolute/path/to/logsl-test
# This can also be set to "kmsg"
StandardOutput=journal
# %N = Full unit name with the type suffix removed
SyslogIdentifier=%N
logsl-test.go
1
2
3
4
5
6
7
8
9
10
11
12
package main
import (
"gopath/to/logsl"
)
func main() {
logsl.Info("Hello, I am Info")
logsl.Warn("Hello, I am Warning")
logsl.Err("Hello, I am Error")
logsl.Fatal("Hello, I am Fatal")
}
logsl.go
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
// A VERY simple logger for systemd daemons.
// @see https://www.srgdev.com/lab/go-syslog
package logsl
import (
"fmt"
"os"
)
// Prints SD_INFO level message to stdout.
func Info(msg ...interface{}) {
os.Stdout.Write([]byte("<6>" + fmt.Sprintln(msg...)))
}
// Prints SD_ERR level message to stdout.
func Err(msg ...interface{}) {
os.Stdout.Write([]byte("<3>ERROR: " + fmt.Sprintln(msg...)))
}
//Prints SD_WARNING level message to stdout.
func Warn(msg ...interface{}) {
os.Stdout.Write([]byte("<4>WARNING: " + fmt.Sprintln(msg...)))
}
// Prints SD_CRIT level message to stdout, followed by a call to panic().
func Panic(msg ...interface{}) {
os.Stdout.Write([]byte("<2>CRITICAL: " + fmt.Sprintln(msg...)))
panic(msg)
}
// Prints SD_ALERT level message to stdout, followed by a call to os.Exit(1).
func Fatal(msg ...interface{}) {
os.Stdout.Write([]byte("<1>FATAL: " + fmt.Sprintln(msg...)))
os.Exit(1)
}